微调一个自己的大模型可能远比想象中更容易
2025-06-14
通过使用WeClone项目,用户可以轻松微调大模型,利用自己的聊天数据创建数字分身,整个过程简单且成本低,只需基础的计算机知识即可上手。微调的关键在于数据,LoRA技术使得在保持原有模型智能的基础上,低成本地优化特定任务表现。
「每日一技」使用patch对npm包缝缝补补
2025-04-17
在前端开发中,使用patch-package或pnpm的内置patch功能可以优雅地修改npm包,保持代码的简便性和持久性。patch-package适合npm和yarn用户,而pnpm提供了更现代的解决方案。
「每日一技」nvm根据项目配置自动切换Node版本
2025-04-07
通过创建 .nvmrc 文件和配置自动切换脚本,可以实现Node.js版本的自动切换,提高开发效率并避免版本不匹配的问题。建议将 .nvmrc 文件提交到版本控制,并在项目README中说明版本要求。
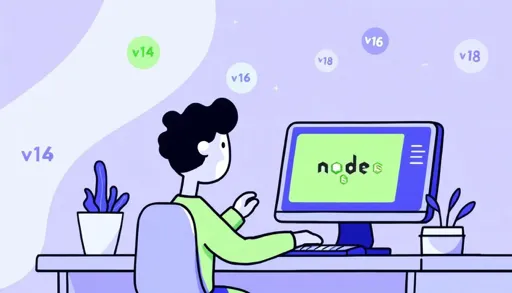
我平时都使用哪些AI工具——Raycast AI开启PC Agent时代
2025-03-17
这篇博客总结了作者在AI工具使用上的经验,特别是如何通过Raycast AI提升工作效率。作者认为AI工具本质上是大脑的延伸,传统工具如Notion等主要解放了双手,而AI工具则进一步减轻了大脑负担。Raycast AI成为作者的主力平台,因其结合本地工具和多模态功能,提供了更强的智能和功能性,超越了之前使用的POE平台。
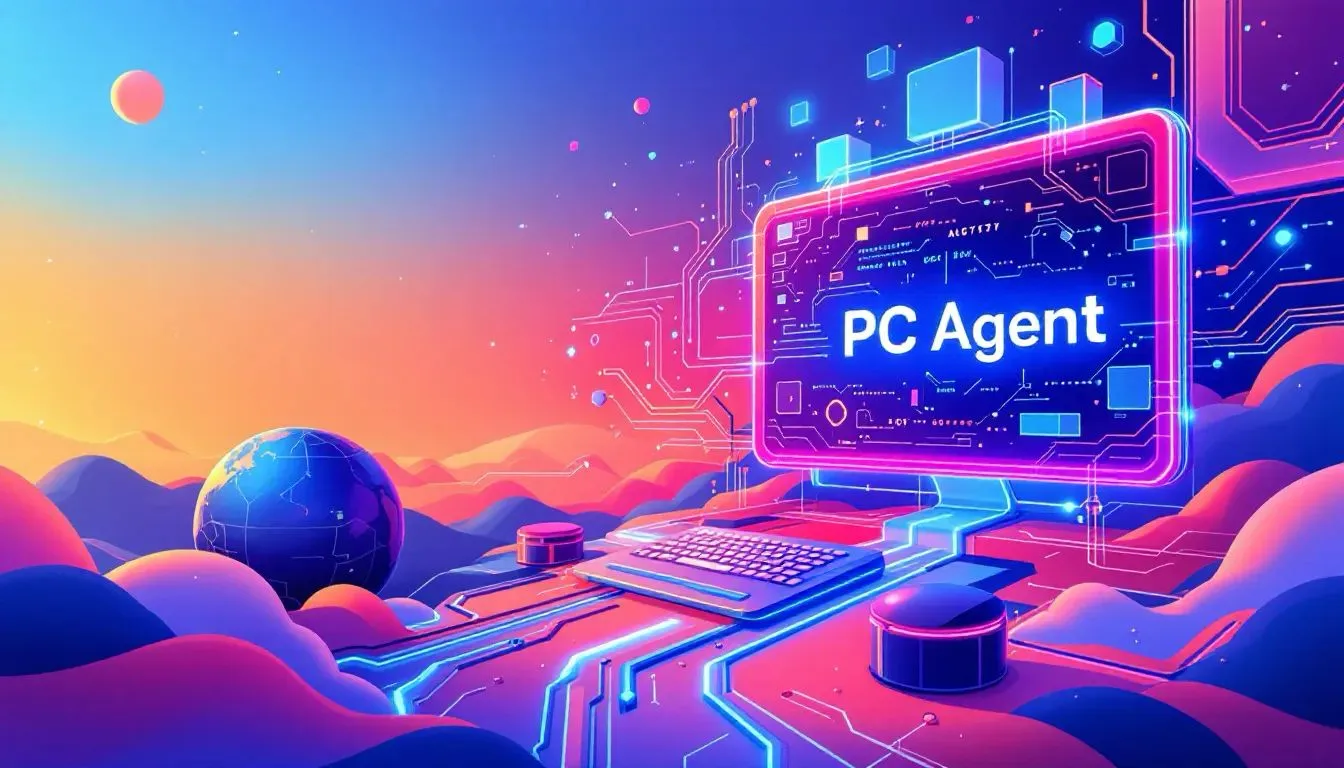
走进与逃离Arc:我的浏览器选择之路
2025-02-19
作者回顾了自己从使用IE到Chrome、Edge,再到Arc浏览器的经历,强调Arc的创新设计和功能,但因性能问题决定寻找替代品,最终选择了Vivaldi浏览器,并通过配置还原Arc的部分体验。
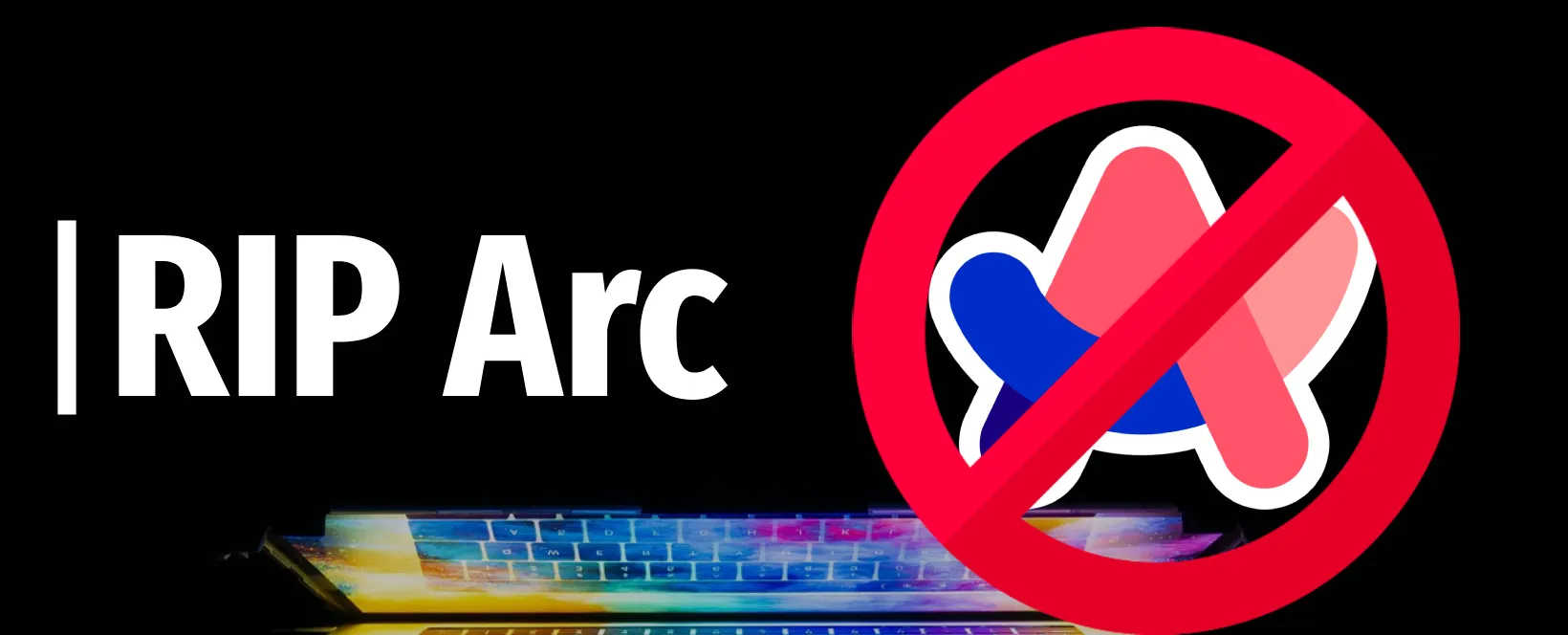
我修好了我的博客——如何设计一个全新的博客框架
2025-02-18
作者分享了个人博客框架迭代历程:从Hexo迁移到Astro的尝试,到发现Elog平台带来的启发,最终思考将Notion作为内容管理系统的新方案。文章重点描述了作者对博客系统的核心需求——解决创作同步问题,以及在探索NotionNext等解决方案过程中遇到的技术限制和思考。
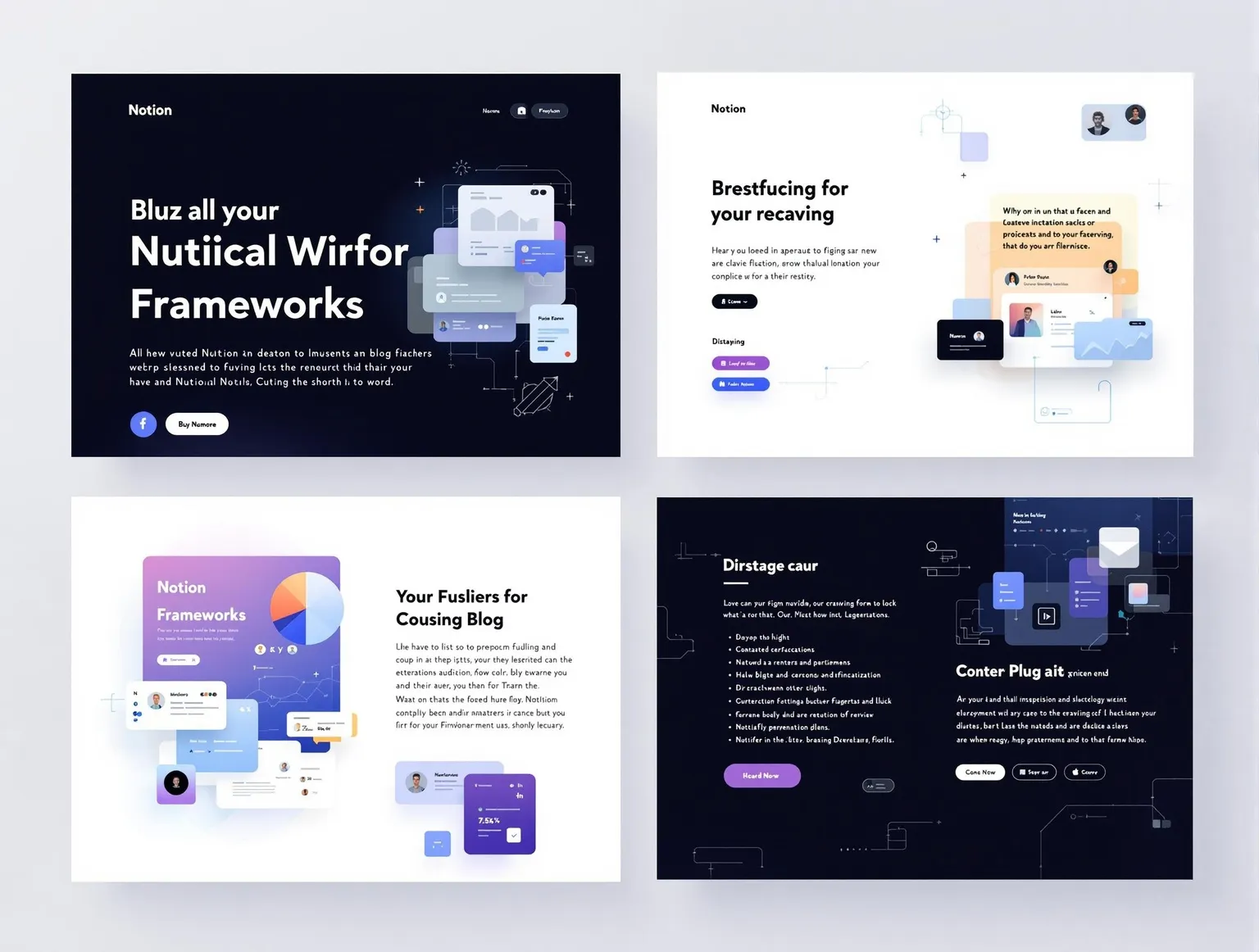
每日一技:VS Code独立配置
2025-01-03
使用VS Code的独立配置功能,可以为不同项目创建特定的配置文件,解决了在公司环境中因安全合规要求而无法使用第三方插件的问题。通过配置云同步和工作区单独配置,满足日常开发需求。推荐使用Codeium作为开源解决方案。
Typescript 类型声明 All-in-one
2025-01-03
TypeScript 的类型声明文件 .d.ts 提供了 JavaScript 代码的附加元信息,支持类型提示和自动补全。使用 DefinitelyTyped 仓库可以为没有类型声明的 npm 包提供类型支持,类型定义可通过 type 和 interface 进行,declare 语法用于为已有的 JS 变量或函数添加类型。三斜杠指令用于引入额外的文件依赖。